【scikit-learn】サンプルデータを取得する方法
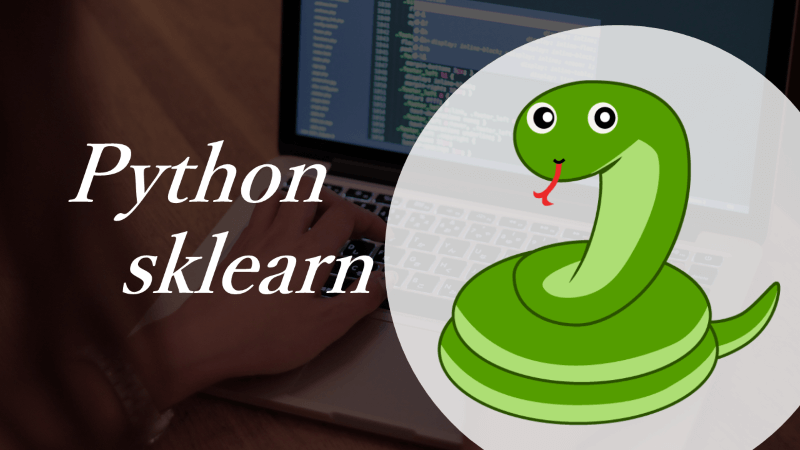
scikit-learnには勉強や練習、テストなどに使えるサンプルデータをロードできる関数が用意されている。
機械学習関連の書籍でも、これらのサンプルデータを対象に色々と処理をしていくことが多い。
何か新しいことをやる場合にはデータサイズや精度的にも割と手ごろな感じとなっている。
回帰用データ
scikit-learnでロードできるデータは、値が全て数値なので、カテゴリー値のエンコーディングが不要。
そのまま計算に使用することができる。
Boston住宅価格データ
みんながお世話になるボストンの住宅価格データはver1.2で廃止となる。
主な理由は、人種差別的な内容がデータから示唆されるため。確かに相関がある。
今後はボストンではなくカリフォルニアの住宅価格データを使うようにする。
California住宅価格データ
ボストン住宅価格データの後継となるデータ。
datasets.fetch_california_housing()で取得できる。
ndarrayとしてデータ取得
引数に何も指定しなければ、ndarrayとして取得される。
計算に用いる場合、ndarrayで取得した方が良いため、通常はこの方法で取得する。
1from sklearn import datasets
2
3# データ取得
4housing = datasets.fetch_california_housing()
5
6# 説明変数と目的変数取得
7X = housing.data
8y = housing.target
9print(f"{X.shape=}, {type(X)=}")
10print(f"{y.shape=}, {type(y)=}")
11
12# X.shape=(20640, 8), type(X)=<class 'numpy.ndarray'>
13# y.shape=(20640,), type(y)=<class 'numpy.ndarray'>
.dataで説明変数を取得できる。.targetで目的変数を取得できる。
ndarrayからDataFrameを作成する
データの特徴を調べていきたい場合、DataFrameにする。
1import pandas as pd
2
3# 目的変数を右端に追加する場合
4df_housing = pd.DataFrame(data=housing.data, columns=housing.feature_names)
5df_housing[housing.target_names[0]] = housing.target
6print(f"{df_housing.shape=}")
7
8# 目的変数を左端に追加する場合
9df_housing = pd.DataFrame(data=housing.data, columns=housing.feature_names)
10df_housing.insert(0, housing.target_names[0], housing.target)
11print(f"{df_housing.shape=}")
12# df_housing.shape=(20640, 9)
.feature_namesで説明変数名のリストを取得できる。.target_namesで目的変数名のリストを取得できる。
1print(df_housing.head())
1>>
2 MedHouseVal MedInc HouseAge AveRooms AveBedrms Population AveOccup \
30 4.526 8.3252 41.0 6.984127 1.023810 322.0 2.555556
41 3.585 8.3014 21.0 6.238137 0.971880 2401.0 2.109842
52 3.521 7.2574 52.0 8.288136 1.073446 496.0 2.802260
63 3.413 5.6431 52.0 5.817352 1.073059 558.0 2.547945
74 3.422 3.8462 52.0 6.281853 1.081081 565.0 2.181467
8
9 Latitude Longitude
100 37.88 -122.23
111 37.86 -122.22
122 37.85 -122.24
133 37.85 -122.25
144 37.85 -122.25
DataFrameとしてデータ取得
DataFrameにしたい場合は、データ取得時に、return_X_yとas_frameをTrueに指定することで、より簡単にDataFrameを作成できる。
1# return_X_yをTrueにすることで、(.data, .target)のタプルが返ってくる。
2# as_frameをTrueにすることで、ndarrayではなくDataFrameが返ってくる。
3df_housing_Xy = datasets.fetch_california_housing(as_frame=True, return_X_y=True)
4# df_housing_Xyはdf_Xとdf_yのタプル
5# 右端の列が目的変数となる
6df_housing = pd.concat(df_housing_Xy, axis=1)
diabetesデータ
糖尿病のデータ。
ndarrayとしてデータ取得
1diabetes = datasets.load_diabetes()
2print(f"{diabetes.data.shape=}")
3print(f"{diabetes.target.shape=}")
4
5# diabetes.data.shape=(442, 10)
6# diabetes.target.shape=(442,)
DataFrameとしてデータ取得
1df_diabetes_Xy = datasets.load_diabetes(return_X_y=True, as_frame=True)
2df_diabetes = pd.concat(df_diabetes_Xy, axis=1)
3print(f"{df_diabetes.shape=}")
4print(df_diabetes.head())
1>>
2df_diabetes.shape=(442, 11)
3 age sex bmi bp s1 s2 s3 \
40 0.038076 0.050680 0.061696 0.021872 -0.044223 -0.034821 -0.043401
51 -0.001882 -0.044642 -0.051474 -0.026328 -0.008449 -0.019163 0.074412
62 0.085299 0.050680 0.044451 -0.005671 -0.045599 -0.034194 -0.032356
73 -0.089063 -0.044642 -0.011595 -0.036656 0.012191 0.024991 -0.036038
84 0.005383 -0.044642 -0.036385 0.021872 0.003935 0.015596 0.008142
9
10 s4 s5 s6 target
110 -0.002592 0.019908 -0.017646 151.0
121 -0.039493 -0.068330 -0.092204 75.0
132 -0.002592 0.002864 -0.025930 141.0
143 0.034309 0.022692 -0.009362 206.0
154 -0.002592 -0.031991 -0.046641 135.0
分類用データ
回帰用データ同様、scikit-learnでロードできるデータは、値が全て数値のみなので、カテゴリー値をエンコーディングする必要がない。
目的変数も最初から数値になっているので、そのまま計算に用いることができる。
breast cancer(乳がん患者)データ
2値分類の基本的なデータ。
ndarrayとしてデータ取得
1breast_cancer = datasets.load_breast_cancer()
2print(f"{breast_cancer.data.shape=}")
3print(f"{breast_cancer.target.shape=}")
4
5# breast_cancer.data.shape=(569, 30)
6# breast_cancer.target.shape=(569,)
30列あるので、不要な特徴量を削る練習に良い。
DataFrameとしてデータ取得
1df_breast_cancer_Xy = datasets.load_breast_cancer(return_X_y=True, as_frame=True)
2df_breast_cancer = pd.concat(df_breast_cancer_Xy, axis=1)
3print(f"{df_breast_cancer.shape=}")
4print(df_breast_cancer.head())
1>>
2df_breast_cancer.shape=(569, 31)
3 mean radius mean texture mean perimeter mean area mean smoothness \
40 17.99 10.38 122.80 1001.0 0.11840
51 20.57 17.77 132.90 1326.0 0.08474
62 19.69 21.25 130.00 1203.0 0.10960
73 11.42 20.38 77.58 386.1 0.14250
84 20.29 14.34 135.10 1297.0 0.10030
9
10 mean compactness mean concavity mean concave points mean symmetry \
110 0.27760 0.3001 0.14710 0.2419
121 0.07864 0.0869 0.07017 0.1812
132 0.15990 0.1974 0.12790 0.2069
143 0.28390 0.2414 0.10520 0.2597
154 0.13280 0.1980 0.10430 0.1809
16
17 mean fractal dimension ... worst texture worst perimeter worst area \
180 0.07871 ... 17.33 184.60 2019.0
191 0.05667 ... 23.41 158.80 1956.0
202 0.05999 ... 25.53 152.50 1709.0
213 0.09744 ... 26.50 98.87 567.7
224 0.05883 ... 16.67 152.20 1575.0
23
24 worst smoothness worst compactness worst concavity worst concave points \
250 0.1622 0.6656 0.7119 0.2654
261 0.1238 0.1866 0.2416 0.1860
272 0.1444 0.4245 0.4504 0.2430
283 0.2098 0.8663 0.6869 0.2575
294 0.1374 0.2050 0.4000 0.1625
30
31 worst symmetry worst fractal dimension target
320 0.4601 0.11890 0
331 0.2750 0.08902 0
342 0.3613 0.08758 0
353 0.6638 0.17300 0
364 0.2364 0.07678 0
37
iris(あやめ)データ
多クラス分類の基本的なデータ。
ndarrayとしてデータ取得
1iris = datasets.load_iris()
2print(f"{iris.data.shape=}")
3print(f"{iris.target.shape=}")
4
5# iris.data.shape=(150, 4)
6# iris.target.shape=(150,)
DataFrameとしてデータ取得
1df_iris_Xy = datasets.load_iris(return_X_y=True, as_frame=True)
2df_iris = pd.concat(df_iris_Xy, axis=1)
3print(f"{df_iris.shape=}")
4print(df_iris.head())
1df_iris.shape=(150, 5)
2 sepal length (cm) sepal width (cm) petal length (cm) petal width (cm) \
30 5.1 3.5 1.4 0.2
41 4.9 3.0 1.4 0.2
52 4.7 3.2 1.3 0.2
63 4.6 3.1 1.5 0.2
74 5.0 3.6 1.4 0.2
8
9 target
100 0
111 0
122 0
133 0
144 0
まとめ
1from sklearn import datasets
2
3# 回帰(住宅価格)
4housing = datasets.fetch_california_housing()
5housing_X = housing.data
6housing_X_names = housing.feature_names
7housing_y = housing.target
8housing_y_names = housing.target_names
9# 各データセットでdata, feature_names, target, target_namesは共通
10
11# 回帰(糖尿病)
12diabetes = datasets.load_diabetes()
13
14# 分類
15# 2値分類
16breast_cancer = datasets.load_breast_cancer()
17# 多クラス分類
18iris = datasets.load_iris()
なお、分類データとして有名なタイタニック号の生存者データはseabornからロードできる。
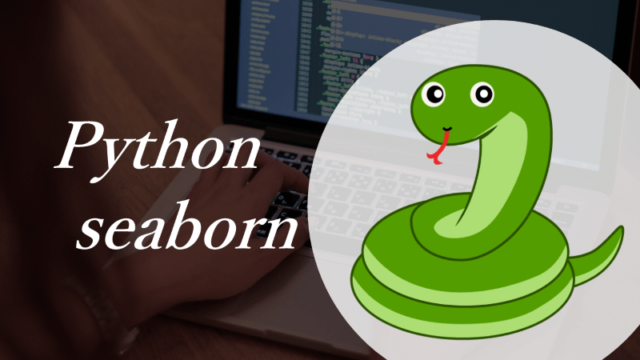
タイタニックデータは、数値以外の値があるため、エンコーディングが必要となるが、目的に合わせて適切なデータセットを選択すると良い。